Google Pay™ - Integration using payform
The steps below will help you setup the Payform with Google Pay in your environment.
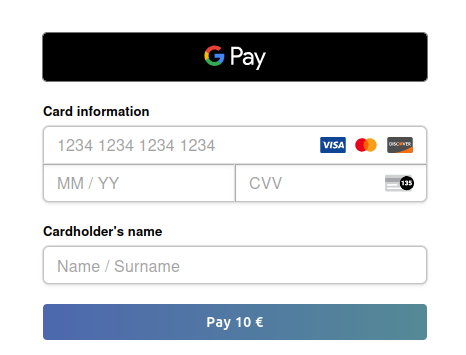
• Step 1: Import EveryPay script
checkout.html
<body>
...
<script src="https://sandbox-js.everypay.gr/v3"></script>
</body>
checkout.html
<body>
...
<script src="https://js.everypay.gr/v3"></script>
</body>
• Step 2: Define the payment element
Create a div
element with id="pay-form"
.
This element is where the form will be positioned within your page.
This element is required by Everypay.js
checkout.html
<body>
<div>
<!-- My App -->
...
<div class="custom-control custom-radio">
<input id="credit" name="paymentMethod" type="radio" class="custom-control-input" onclick="everypay.showForm()" required>
<label class="custom-control-label" for="credit">Credit card</label>
<div id="pay-form"></div>
</div>
...
</div>
</body>
• Step 3: Mount the form
Let's create the previously declared function in our scripts section.
Now lets call the everypay.payform()
function.
The everypay.payform()
function expects a payload and a response handler.
Here you can read more about the Payload that is available.
checkout.html
...
<script>
var payload = {
pk: 'your-public-key',
amount: 1000,
locale: 'el',
data: {
email: "my_customer@example.com"
},
otherPaymentMethods: {
googlePay: {
countryCode: "GR",
merchantName: "MerchantName",
merchantUrl: "https://www.merchant-url.com/",
allowedCardNetworks: ["VISA", "MASTERCARD"],
allowedAuthMethods: ["CRYPTOGRAM_3DS", "PAN_ONLY"],
},
},
}
function handleResponse(r) {
if (r.response === 'success') {
// You have the token! Submit it to your backend in order to charge the card
axios.post("https://mybackend/endpoint", {
token: r.token
})
}
else {
// Inform the user if there was an error
}
}
everypay.payform(payload, handleResponse);
</script>
• Step 4: Charge the card
Now that you have the token in your backend you can use it to charge the card. To do that use the /payments endpoint in our api